Requirements: Echo with "Trigger Command" Alexa Skill, TriggerCMD installed on desired computer, Nodejs - I recently upgraded to 8.9.3 - Just make sure you're not far behind on your installed Nodejs, I'm using Windows 10 - I believe you can get selenium-webdriver for MAC and LINUX.
LINK FOR REFERENCE: http://seleniumhq.github.io/selenium/docs/api/javascript/
INSTRUCTIONS
- Open https://www.npmjs.com/package/selenium-webdriver
- Open windows command prompt
- cd to desired directory (I'm a web developer) so htdocs folder for me THEN type npm install selenium-webdriver.
- Once selenium-webdriver is installed you may need to install additional components - There are links/downloads for these components in my LINK FOR REFERENCE above. I did not need to install this for Firefox. I guess I already had this installed. (If you need this the link is just to download whichever browsers developer browser executable to use with selenium. You will need to add a PATH variable to the directory of the executables.)
- Open your IDE of choice and navigate to node_modules->selenium-webdrivers->examples->google_search.js. Try running this. You can do so by running the script from the command line->
node C:\MAMP\htdocs\automation\node_modules\selenium-webdriver\example>node google_search.js
If your component was installed correctly this will open up your browser and do a google search. Then you know its working and you can use my code below to have Alexa open up a specific movie.
// or more contributor license agreements. See the NOTICE file
// distributed with this work for additional information
// regarding copyright ownership. The SFC licenses this file
// to you under the Apache License, Version 2.0 (the
// "License"); you may not use this file except in compliance
// with the License. You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing,
// software distributed under the License is distributed on an
// "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
// KIND, either express or implied. See the License for the
// specific language governing permissions and limitations
// under the License.
/**
* @fileoverview An example WebDriver script.
*
* Before running this script, ensure that Mozilla's geckodriver is present on
* your system PATH: <https://github.com/mozilla/geckodriver/releases>
*
* Usage:
* // Default behavior
* node selenium-webdriver/example/google_search.js
*
* // Target Chrome locally; the chromedriver must be on your PATH
* SELENIUM_BROWSER=chrome node selenium-webdriver/example/google_search.js
*
* // Use a local copy of the standalone Selenium server
* SELENIUM_SERVER_JAR=/path/to/selenium-server-standalone.jar \
* node selenium-webdriver/example/google_search.js
*
* // Target a remote Selenium server
* SELENIUM_REMOTE_URL=http://www.example.com:4444/wd/hub \
* node selenium-webdriver/example/google_search.js
*/
const {Builder, By, Key, until} = require('..');
var driver = new Builder()
.forBrowser('firefox')
.build();
var command = "";
///GETTING COMMAND FROM AGRUMENTS
process.argv.map((value, index) => {
if(index > 2) {
return command = command + " " + value;
} else if( index === 2) {
return command = value;
}
});
// .then(_ => driver.wait(until.titleIs('webdriver - Google Search'), 1000))
driver.get('https://www.netflix.com/login')
.then(_ =>
driver.findElement(By.name('email')).sendKeys('netflixuser@gmail.com'))
.then(_ =>
driver.findElement(By.name('password')).sendKeys('netflixpassword'), Key.ENTER)
.then(_ =>
driver.sleep(2000))
.then(_ =>
driver.findElement(By.className('login-button')).click())
.then(_ =>
driver.sleep(1000))
.then(_ =>
driver.findElement(By.xpath('/html/body/div[1]/div/div/div[2]/div/div/ul/li[1]/div/a/div/div')).click())
.then(_ =>
driver.findElement(By.className('searchTab')).click())
.then(_ =>
driver.findElement(By.css('.searchInput > input:nth-child(2)')).sendKeys(command))
.then(_ =>
driver.sleep(3000))
.then(_ =>
driver.findElement(By.id('title-card-0-0')).click())
.then(_ =>
driver.sleep(2000))
.then(_ =>
driver.findElement(By.className('play')).click())
.then(_ =>
driver.sleep(2000))
.then(_ =>
driver.findElement(By.css('body')).click());
- Run Test with Netflix script -> Lets say you want to just copy my code and replace the google_search.js file you tested with. In your command prompt cd to your selenium-webdriver->example directory and in the command prompt type "node google_search.js boss baby and press enter. This will run the script and open up boss baby.
Here's mine.
C:\MAMP\htdocs\automation\node_modules\selenium-webdriver\example>node google_search.js boss baby
So, "boss baby" is the parameter that you are going to pass to "Alexa". Once you have the Alexa skill enabled and the script working just say the command you created. Such as: "Alexa open trigger command and movie with parameter boss baby"
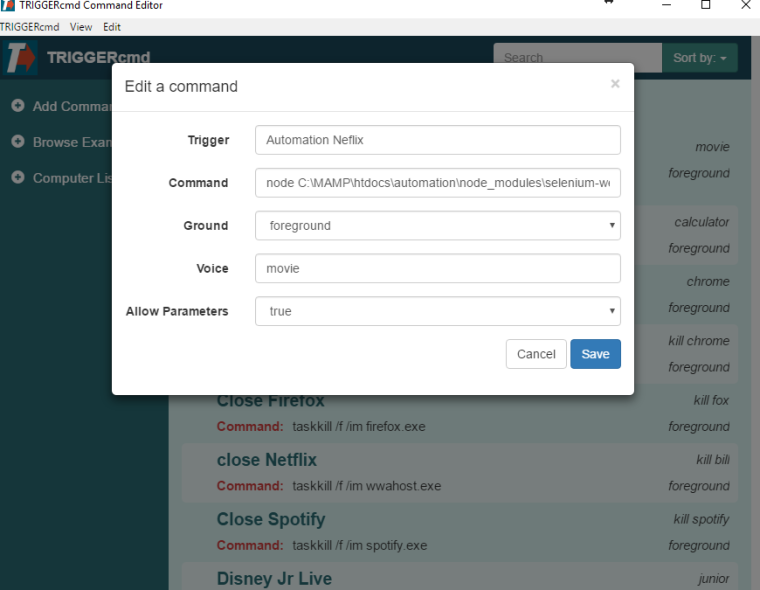
I realize you cannot see my full command its just the same path as the google_search.js script above - C:\MAMP\htdocs\automation\node_modules\selenium-webdriver\example>node google_search.js
If you have any troubles with this let me know. I have noticed that there are times when the script fails because it says that an element on the page isn't there yet. You can increase the sleep time on the script. Like instead of 2 seconds (2000) change to three (3000). Now you can start building your own scripts. The driver class methods being used has helpful comments in the C:\MAMP\htdocs\automation\node_modules\selenium-webdriver\lib\webdriver.js file. I used this to learn how to change up the script to my needs (Netflix by specific movie). My kid loves it!
I split video in two to cut out my login/users page - but this is how it works for me.
https://drive.google.com/file/d/1_V-5zjNurB_a-DyaBgNE4CljzUBhhqLW/view?usp=sharing
https://drive.google.com/file/d/1t82l-Y6Tb5JLhKpMElxP4YRD1Ta7Z7TL/view?usp=sharing